mirror of
https://github.com/rizinorg/cutter.git
synced 2024-12-19 11:26:11 +00:00
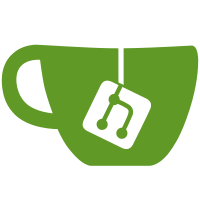
* Update deps scripts for macOS * Update deps * Update appbundle_embed_python.sh * Use cutter-deps for macOS * Remove qt from Brewfile * Fix .travis.yml * Disable Homebrew update * Fix llvm for macOS * Fix regex in update_deps.py * Fix PYTHON_FRAMEWORK_DIR * Some debug output in travis * Enable Python Bindings for cmake on macOS * QMake debug * Update deps * Hopefully fix pkg-config * QMake hack for shiboken2 and pyside2 * Deploy on deps-test * Update deps * Fix call to appbundle_embed_python.sh * Update deps * Fix Python for cmake on macOS * Update deps * Fix appbundle_embed_python.sh * Copy pyside libs in appbundle_embed_python.sh * Fix includes for CMake on macOS * Copy PySide2 and Shiboke2 libs to Frameworks dir * Manually deploy QtDBus and QtPrintSupport * Print plugins dir * Fix appbundle_embed_python.sh * Update deps * Remove some debug output * Use last release of linuxdeployqt
60 lines
1.4 KiB
C
60 lines
1.4 KiB
C
/** \file CutterCommon.h
|
|
* This file contains any definition that is useful in the whole project.
|
|
* For example, it may contain custom types (RVA, ut64), list iterators, etc.
|
|
*/
|
|
#ifndef CUTTERCORE_H
|
|
#define CUTTERCORE_H
|
|
|
|
#include "r_core.h"
|
|
#include <QString>
|
|
|
|
// Workaround for compile errors on Windows
|
|
#ifdef _WIN32
|
|
#undef min
|
|
#undef max
|
|
#endif //_WIN32
|
|
|
|
// radare2 list iteration macros
|
|
#define CutterRListForeach(list, it, type, x) \
|
|
if (list) for (it = list->head; it && ((x=static_cast<type*>(it->data))); it = it->n)
|
|
|
|
#define CutterRVectorForeach(vec, it, type) \
|
|
if ((vec) && (vec)->a) \
|
|
for (it = (type *)(vec)->a; (char *)it != (char *)(vec)->a + ((vec)->len * (vec)->elem_size); it = (type *)((char *)it + (vec)->elem_size))
|
|
|
|
// Global information for Cutter
|
|
#define APPNAME "Cutter"
|
|
|
|
/**
|
|
* @brief Type to be used for all kinds of addresses/offsets in r2 address space.
|
|
*/
|
|
typedef ut64 RVA;
|
|
|
|
/**
|
|
* @brief Maximum value of RVA. Do NOT use this for specifying invalid values, use RVA_INVALID instead.
|
|
*/
|
|
#define RVA_MAX UT64_MAX
|
|
|
|
/**
|
|
* @brief Value for specifying an invalid RVA.
|
|
*/
|
|
#define RVA_INVALID RVA_MAX
|
|
|
|
inline QString RAddressString(RVA addr)
|
|
{
|
|
return QString::asprintf("%#010llx", addr);
|
|
}
|
|
|
|
inline QString RSizeString(RVA size)
|
|
{
|
|
return QString::asprintf("%lld", size);
|
|
}
|
|
|
|
inline QString RHexString(RVA size)
|
|
{
|
|
return QString::asprintf("%#llx", size);
|
|
}
|
|
|
|
#endif // CUTTERCORE_H
|
|
|